Gtk RadioButton
Setting up the Python
First we set up the minumum Python to load our glade and shut down properly. We must define functions for every signal handler we created in the glade file. For now we just have functions that do nothing with the pass except for the function that is connected to window1 destroy handler on_window1_destroy.
#!/usr/bin/env python
import gtk
class main:
def __init__(self):
self.builder = gtk.Builder()
self.builder.add_from_file('gtk11.glade')
self.builder.connect_signals(self)
self.window = self.builder.get_object('window1')
self.window.show()
def button_toggle(self, widget):
pass
def on_window1_destroy(self, object, data=None):
print "quit with cancel"
gtk.main_quit()
if __name__ == "__main__":
main = main()
gtk.main()
Save the Python file as gtk11.py and set the permission to execute.
When you run gtk11.py your project should look like this:

At this point the only thing that works is if you click on the X the window will politely go away. I like to start with a working code base so I know at least the basic part works as I add to the code.
Handling the Toggled Signal
Each time a radio button changes states the toggled signal is emitted. This means that when you press a radio button the one you selected and the one that turned off both emit the toggled signal. To determine which is which we use the widget.get_active() function. Lets look at the complete button_toggle function. When the radio button is toggled it calls the button_toggle function and passes along the widget. The widget can now be referenced. The first line we check to see if the radio button that triggered the toggled signal is active which means it is the selected one. In the second line we check to see if the radio button that triggered the toggled signal is not active which means it is not the selected one.
def button_toggle(self, widget):
if widget.get_active():print 'active ' + gtk.Buildable.get_name(widget)
if not widget.get_active():print 'active ' + gtk.Buildable.get_name(widget)
When we add this to our code and run gtk11.py we see the print to the terminal each radio button that triggered the toggled signal.
When you run gtk11.py your project should look like this:
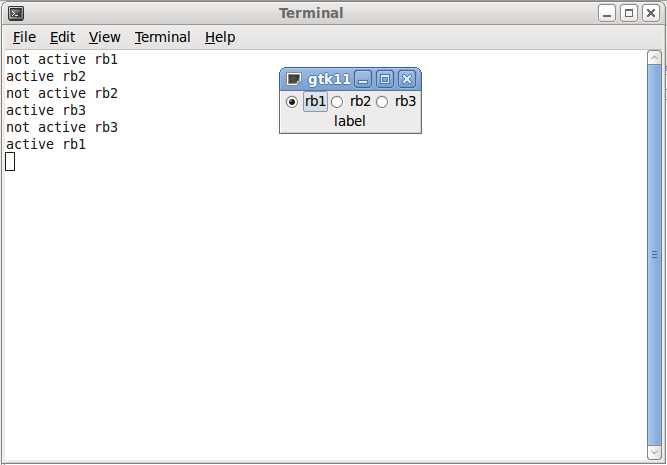
If you notice in the terminal window I pressed rb2 and the first two lines were printed showing that rb1 is not active and rb2 is now active. The rb3 radio button didn’t change states so it did not fire the toggled signal. By using get_active() and gtk.Buildable.get_name(widget) we can tell which radio button is the current active one and know it’s name all in one little function.
Reacting to a Toggled Signal
Now that we can tell which radio button is the active one lets do something based on which button is the active one.
In the __init__ lets add one line.
self.builder.connect_signals(self)
self.label1 = self.builder.get_object('label1')
self.window = self.builder.get_object('window1')
Now lets change the button_toggle function to change the text of the label to match the selected radio button. We are only interested in the currently selected radio button so we us the if widget.get_active(): to screen out the radio button that is not selected. Then we use the the gtk.Buildable.get_name(widget) to find out which one is active and then run our code based on that information.
def button_toggle(self, widget):
if widget.get_active():
# if the active widget name is equal to 'rb1': then set the label text
if gtk.Buildable.get_name(widget)=='rb1':self.label1.set_text('rb1 is selected')
if gtk.Buildable.get_name(widget)=='rb2':self.label1.set_text('rb2 is selected')
if gtk.Buildable.get_name(widget)=='rb3':self.label1.set_text('rb3 is selected')
Now when we run the program the label shows the selected radio button.

The files used in this tutorial: