Gtk Entry Widget
The Python
We made some changes to the glade3.py file, the first one we imported the math library so we can use the math.pi constant. We could have just typed the number in but it is better to learn how to import a library and use a constant. So after importing the other libraries we add the following code.
try:
import math
except:
print('math lib missing')
sys.exit(1)
The next thing we added to our code is the define that handles what happens when we click on the Calc button. For brevity no error checking has been done to make sure the entries in the GtkEntry widget are correct.
def on_sfm_button_clicked(self, button, data=None):
# create an instance of the entry objects
# so we can get and set the text values
self.entry1 = self.builder.get_object("entry1")
self.entry2 = self.builder.get_object("entry2")
self.result1 = self.builder.get_object("result1")
# get the text from the GtkEntry widget and convert
# it to a float value so we can calculate the result
self.sfm = float(self.entry1.get_text())
self.diameter = float(self.entry2.get_text())
# calculate the result convert to an int to round the number
# then convert to a string to set the text in our label
# notice the math.pi constant is used in the calculation
self.rpm = str(int(self.sfm * ((12/math.pi)/self.diameter)))
# debugging print
print "calculate rpm clicked"
# set the result label with our results
self.result1.set_text(self.rpm)
The following is the complete python file.
#!/usr/bin/env python
import gtk
import math
class Buglump:
def __init__(self):
self.gladefile = "glade4.glade"
self.builder = gtk.Builder()
self.builder.add_from_file(self.gladefile)
self.builder.connect_signals(self)
self.window = self.builder.get_object("window1")
self.aboutdialog = self.builder.get_object("aboutdialog1")
self.statusbar = self.builder.get_object("statusbar1")
self.context_id = self.statusbar.get_context_id("status")
self.status_count = 0
self.window.show()
def on_window1_destroy(self, object, data=None):
print "quit with cancel"
gtk.main_quit()
def on_gtk_quit_activate(self, menuitem, data=None):
print "quit from menu"
gtk.main_quit()
def on_push_status_activate(self, menuitem, data=None):
self.status_count += 1
self.statusbar.push(self.context_id, "Message number %s" % str(self.status_count))
def on_pop_status_activate(self, menuitem, data=None):
self.status_count -= 1
self.statusbar.pop(self.context_id)
def on_clear_status_activate(self, menuitem, data=None):
while (self.status_count > 0):
self.statusbar.pop(self.context_id)
self.status_count -= 1
def on_gtk_about_activate(self, menuitem, data=None):
print "help about selected"
self.response = self.aboutdialog.run()
self.aboutdialog.hide()
def on_sfm_button_clicked(self, button, data=None):
self.entry1 = self.builder.get_object("entry1")
self.entry2 = self.builder.get_object("entry2")
self.result1 = self.builder.get_object("result1")
self.sfm = float(self.entry1.get_text())
self.diameter = float(self.entry2.get_text())
self.rpm = str(int(self.sfm * ((12/math.pi)/self.diameter)))
print "calculate rpm clicked"
self.result1.set_text(self.rpm)
if __name__ == "__main__":
main = Buglump()
gtk.main()
When we run the program it looks like the following image. You can enter numbers in each GtkEntry widget and press the Calc button and see the result of your calculation.
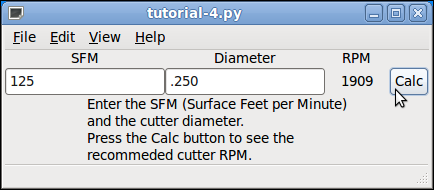
That’s all for this one and we still didn’t even say Hello World… but we actually did something useful which is amazing.